import javax.xml.parsers.*;
import org.xml.sax.InputSource;
import org.w3c.dom.*;
import java.io.*;
public class ParseXMLString {
public static void main(String arg[]) {
String xmlRecords =
"<data>" +
" <employee>" +
" <name>John</name>" +
" <title>Manager</title>" +
" </employee>" +
" <employee>" +
" <name>Sara</name>" +
" <title>Clerk</title>" +
" </employee>" +
"</data>";
try {
DocumentBuilderFactory dbf =
DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
InputSource is = new InputSource();
is.setCharacterStream(new StringReader(xmlRecords));
Document doc = db.parse(is);
NodeList nodes = doc.getElementsByTagName("employee");
// iterate the employees
for (int i = 0; i < nodes.getLength(); i++) {
Element element = (Element) nodes.item(i);
NodeList name = element.getElementsByTagName("name");
Element line = (Element) name.item(0);
System.out.println("Name: " + getCharacterDataFromElement(line));
NodeList title = element.getElementsByTagName("title");
line = (Element) title.item(0);
System.out.println("Title: " + getCharacterDataFromElement(line));
}
}
catch (Exception e) {
e.printStackTrace();
}
/*
output :
Name: John
Title: Manager
Name: Sara
Title: Clerk
*/
}
public static String getCharacterDataFromElement(Element e) {
Node child = e.getFirstChild();
if (child instanceof CharacterData) {
CharacterData cd = (CharacterData) child;
return cd.getData();
}
return "?";
}
}
Friday, May 29, 2009
Parse an XML string
Add the Google Analytics Code Block to Your Blogger Blog
1. Login to http://www.blogger.com/. The Dashboard loads.
2. Under the blog you want to add Analytics tracking to, click on Layout or Template.
3. Click on Edit HTML. An editing screen for your blog template's HTML displays. Don't freak out. Just scroll to the bottom.
4. Look for the end of the template. It'll look like:
</div> </div>
<!-- end outer-wrapper -->
(Google Analytics Code Block is going to go here!!!)
</body>
</html>
5. Put your cursor right before that </body> tag.
6. Paste the Google Analytics Code Block by selecting Edit > Paste, Ctrl -V or Command-V.
7. Click Save Changes.
http://andywibbels.com/2007/01/how-to-add-google-analytics-to-your-blogger-blog/
2. Under the blog you want to add Analytics tracking to, click on Layout or Template.
3. Click on Edit HTML. An editing screen for your blog template's HTML displays. Don't freak out. Just scroll to the bottom.
4. Look for the end of the template. It'll look like:
</div> </div>
<!-- end outer-wrapper -->
(Google Analytics Code Block is going to go here!!!)
</body>
</html>
5. Put your cursor right before that </body> tag.
6. Paste the Google Analytics Code Block by selecting Edit > Paste, Ctrl -V or Command-V.
7. Click Save Changes.
http://andywibbels.com/2007/01/how-to-add-google-analytics-to-your-blogger-blog/
Thursday, May 28, 2009
Soap Action and Addressing action
In WS-I version 1.1 it states
The SOAPAction header is purely a hint to processors. All vital information regarding the intent of a message is carried in the envelope.
– `A HTTP request MESSAGE MUST contain a SOAPAction HTTP header field with a quoted value equal to the value of the soapAction attribute of soapbind:operation, if present in the corresponding WSDL description.`
– `A HTTP request MESSAGE MUST contain a SOAPAction HTTP header field with a quoted empty string value, if in the corresponding WSDL description, the soapAction of soapbind:operation is either not present, or present with an empty string as its value.`
Again the `Web Services Addressing 1.0 – WSDL Binding` states clearly about the explicit association between wsa:action and SOAPAction as follows
`WS-Addressing defines a global attribute, wsaw:Action, that can be used to explicitly define the value of the [action] property for messages in a WSDL description. The type of the attribute is xs:anyURI and it is used as an extension on the WSDL input, output and fault elements. A SOAP binding can specify SOAPAction values for the input messages of operations. In the absence of a wsaw:Action attribute on a WSDL input element where a SOAPAction value is specified, the value of the [action] property for the input message is the value of the SOAPAction specified. Web Services Addressing 1.0 – SOAP Binding[WS-Addressing SOAP Binding] specifies restrictions on the relationship between the values of [action] and SOAPAction for SOAP 1.1 and SOAP 1.2.
The inclusion of wsaw:Action without inclusion of wsaw:UsingAddressing has no normative intent and is only informational. In other words, the inclusion of wsaw:Action attributes in WSDL alone does not imply a requirement on clients to use Message Addressing Properties in messages it sends to the service. A client, however, MAY include Message Addressing Properties in the messages it sends, either on its own initiative or as described by other elements of the service contract, regardless of the presence or absence of wsaw:UsingAddressing. Other specifications defining the value of [action] are under no constraint to be consistent with wsaw:Action.`
http://damithakumarage.wordpress.com/2008/02/12/soap-action-and-addressing-action/
The SOAPAction header is purely a hint to processors. All vital information regarding the intent of a message is carried in the envelope.
– `A HTTP request MESSAGE MUST contain a SOAPAction HTTP header field with a quoted value equal to the value of the soapAction attribute of soapbind:operation, if present in the corresponding WSDL description.`
– `A HTTP request MESSAGE MUST contain a SOAPAction HTTP header field with a quoted empty string value, if in the corresponding WSDL description, the soapAction of soapbind:operation is either not present, or present with an empty string as its value.`
Again the `Web Services Addressing 1.0 – WSDL Binding` states clearly about the explicit association between wsa:action and SOAPAction as follows
`WS-Addressing defines a global attribute, wsaw:Action, that can be used to explicitly define the value of the [action] property for messages in a WSDL description. The type of the attribute is xs:anyURI and it is used as an extension on the WSDL input, output and fault elements. A SOAP binding can specify SOAPAction values for the input messages of operations. In the absence of a wsaw:Action attribute on a WSDL input element where a SOAPAction value is specified, the value of the [action] property for the input message is the value of the SOAPAction specified. Web Services Addressing 1.0 – SOAP Binding[WS-Addressing SOAP Binding] specifies restrictions on the relationship between the values of [action] and SOAPAction for SOAP 1.1 and SOAP 1.2.
The inclusion of wsaw:Action without inclusion of wsaw:UsingAddressing has no normative intent and is only informational. In other words, the inclusion of wsaw:Action attributes in WSDL alone does not imply a requirement on clients to use Message Addressing Properties in messages it sends to the service. A client, however, MAY include Message Addressing Properties in the messages it sends, either on its own initiative or as described by other elements of the service contract, regardless of the presence or absence of wsaw:UsingAddressing. Other specifications defining the value of [action] are under no constraint to be consistent with wsaw:Action.`
http://damithakumarage.wordpress.com/2008/02/12/soap-action-and-addressing-action/
Wednesday, May 27, 2009
Alexa Internet
Alexa Internet, Inc. is a California-based subsidiary company of Amazon.com that is known for its toolbar and website. Once installed, the toolbar collects data on browsing behavior which is transmitted to the website where it is stored and analyzed and is the basis for the company's web traffic reporting.
http://alexa.com/
http://alexa.com/
Sunday, May 24, 2009
City Data
Stats about all US cities - real estate, relocation info, house prices, home value estimator, recent sales, maps, race, income, photos, education, crime, ...
http://www.city-data.com/
http://www.city-data.com/
Friday, May 22, 2009
Difference between JVM and JRE
A JVM is just the "bare machine" -- the thing that executes .class files.
A JRE includes libraries and APIs (i.e., all the java.* and javax.* Java classes, plus things like the native libaries needed for the AWT to function.) So a JRE is a JVM plus more stuff.
http://www.coderanch.com/t/416610/Java-General-beginner/java/Difference-between-JVM-JRE
A JRE includes libraries and APIs (i.e., all the java.* and javax.* Java classes, plus things like the native libaries needed for the AWT to function.) So a JRE is a JVM plus more stuff.
http://www.coderanch.com/t/416610/Java-General-beginner/java/Difference-between-JVM-JRE
Understanding the Difference Between an Argument and a Parameter
The words argument and parameter are often used interchangeably in the literature, although the C++ Standard makes a clear distinction between the two. An argument is one of the following: an expression in the comma-separated list in a function call; a sequence of one or more preprocessor tokens in the comma-separated list in a macro call; the operand of a throw-statement or an expression, type, or template-name in the comma-separated list in the angle brackets of a template instantiation. A parameter is one of the following: an object or reference that is declared in a function declaration or definition (or in the catch clause of an exception handler); an identifier between the parentheses immediately following the macro name in a macro definition; or a template-parameter. This example demonstrates the difference between a parameter and an argument:
void func(int n, char * pc); //n and pc are parameters
template class A {}; //T is a a parameter
http://www.devx.com/tips/Tip/13049
void func(int n, char * pc); //n and pc are parameters
template
int main()
{
char c;
char *p = &c;
func(5, p); //5 and p are arguments
Aa; //'long' is an argument
Aanother_a; //'char' is an argument
return 0;
}
http://www.devx.com/tips/Tip/13049
Wednesday, May 20, 2009
Activity state changes
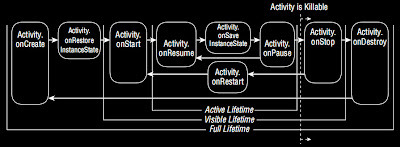
State change method handlers available in an Activity
package com.paad.myapplication;
import android.app.Activity;
import android.os.Bundle;
public class MyActivity extends Activity {
// Called at the start of the full lifetime.
@Override
public void onCreate(Bundle icicle) {
super.onCreate(icicle);
// Initialize activity.
}
// Called after onCreate has finished, use to restore UI state
@Override
public void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
// Restore UI state from the savedInstanceState.
// This bundle has also been passed to onCreate.
}
// Called before subsequent visible lifetimes
// for an activity process.
@Override
public void onRestart(){
super.onRestart();
// Load changes knowing that the activity has already
// been visible within this process.
}
// Called at the start of the visible lifetime.
@Override
public void onStart(){
super.onStart();
// Apply any required UI change now that the Activity is visible.
}
// Called at the start of the active lifetime.
@Override
public void onResume(){
super.onResume();
// Resume any paused UI updates, threads, or processes required
// by the activity but suspended when it was inactive.
}
// Called to save UI state changes at the
// end of the active lifecycle.
@Override
public void onSaveInstanceState(Bundle savedInstanceState) {
// Save UI state changes to the savedInstanceState.
// This bundle will be passed to onCreate if the process is
// killed and restarted.
super.onSaveInstanceState(savedInstanceState);
}
// Called at the end of the active lifetime.
@Override
public void onPause(){
// Suspend UI updates, threads, or CPU intensive processes
// that don’t need to be updated when the Activity isn’t
// the active foreground activity.
super.onPause();
}
// Called at the end of the visible lifetime.
@Override
public void onStop(){
// Suspend remaining UI updates, threads, or processing
// that aren’t required when the Activity isn’t visible.
// Persist all edits or state changes
// as after this call the process is likely to be killed.
super.onStop();
}
// Called at the end of the full lifetime.
@Override
public void onDestroy(){
// Clean up any resources including ending threads,
// closing database connections etc.
super.onDestroy();
}
}
Tuesday, May 19, 2009
Differences between @+id/foo and @android:id/foo syntaxes in main.xml
@+id/foo means you are creating an id named foo in the namespace of your application. You can refer to it using @id/foo.
@android:id/foo means you are referring to an id defined in the android namespace.
This namespace is the namespace of the framework. In this case, you need to use @android:id/list and @android:id/empty because these are the id the framework expects to find (the framework knows only about the ids in the android namespace.)
http://groups.google.com/group/android-developers/browse_thread/thread/dc8023b221351aa7?pli=1
@android:id/foo means you are referring to an id defined in the android namespace.
This namespace is the namespace of the framework. In this case, you need to use @android:id/list and @android:id/empty because these are the id the framework expects to find (the framework knows only about the ids in the android namespace.)
http://groups.google.com/group/android-developers/browse_thread/thread/dc8023b221351aa7?pli=1
Saturday, May 16, 2009
Import Android project into Eclipse
- Create new workspace in Eclipse (optional) anywhere OUTSIDE of the folder that contains project you are importing
- Click File -> New -> Android Project
- From the pop-up select “Create project from existing source” and navigate to the top folder of Android project you are importing
- Click “Finish”
Thursday, May 14, 2009
CDYNE Web Service WIKI
CDYNE Corporation develops, markets and supports a comprehensive suite of data enhancement, data quality and data analysis Web services and business intelligence integration. These Web services are ideal for enterprise database systems: home-grown applications in the E-commerce, Telecommunications, Sales/Marketing, Insurance, Retail, Utilities, Health care, and Banking/Finance Industries across the United States. CDYNE A leading provider of Services & Solutions for business intelligence, data cleansing, and standards-based integration in Internet/Intranet software applications. Addionly an ERP (Enterprise Resource Planning) & CRM (Customer Relationship Management) systems. Our Web services' allow organizations to make more informed intelligent decisions; as well as drive bottom-line sales and marketing efforts that ultimately lead to the more efficient use of corporate resources. CDYNE delivers the lowest cost of ownership, swift uptime results, consistent enterprise data and high-performance Web services' that "works" the way 'we think. CDYNE Technologies are built on proven XML-based information services that dynamically deliver value-added information at the point of consumer interaction. A Captured "real-time service" for the ultimate in data delivery and integration efficiency. With end-to-end, fully integrated data movement, access and analysis capabilities on any database platform, it is capable of operating in standalone/custom applications websites or CRM/ERP solutions. As a result, our Web Service Technologies enables organizations to significantly improve customer satisfaction, minimize service costs, and increase revenue. CDYNE’s principle offices are located in Chesapeake, Virginia. This facility serves as an administration and redundant server site facility. Our solutions are delivered utilizing state-of-the-art software and hardware architecture operating in 24x7 computing facilities.
http://wiki.cdyne.com/wiki/index.php?title=CDYNE_Wiki_for_Web_Services:About
http://wiki.cdyne.com/wiki/index.php?title=CDYNE_Wiki_for_Web_Services:About
Android Market vs. iPhone App Store: The First 24 Hours
Google’s Android Market has been officially live for 24 hours. Here are some early observations and comparisons with the iPhone App Store’s first 24 hours.
There are myriad similarities between iPhone and Android users:
* They like to play games, shop, and know what music they are listening to,
* They are curious about the weather, and
* They generally share the same interests as iPhone users
During the first 24 hours of Android Market, 62 apps were available to consumers, all free. This is less than 10% of the number of apps we saw at the launch of Apple’s App Store. Although Apple allowed both free and paid applications to be distributed when the App Store launched, paid downloads for Android will not be available until Q1 2009.
http://www.medialets.com/blog/2008/10/23/android-market-vs-iphone-app-store-the-first-24-hours/
There are myriad similarities between iPhone and Android users:
* They like to play games, shop, and know what music they are listening to,
* They are curious about the weather, and
* They generally share the same interests as iPhone users
During the first 24 hours of Android Market, 62 apps were available to consumers, all free. This is less than 10% of the number of apps we saw at the launch of Apple’s App Store. Although Apple allowed both free and paid applications to be distributed when the App Store launched, paid downloads for Android will not be available until Q1 2009.
http://www.medialets.com/blog/2008/10/23/android-market-vs-iphone-app-store-the-first-24-hours/
Monday, May 11, 2009
Taskbar Shuffle Reorganizes Your Taskbar and System Tray
Windows only: Tiny, portable, and awesome utility Taskbar Shuffle adds drag and drop ability to the Windows taskbar and system tray—and now finally works for 64-bit Windows as well.
http://lifehacker.com/5239479/taskbar-shuffle-reorganizes-your-taskbar-and-system-tray#c12590263
http://lifehacker.com/5239479/taskbar-shuffle-reorganizes-your-taskbar-and-system-tray#c12590263
Monday, May 4, 2009
Add National & Religious Holidays to Google Calendar
Just like Microsoft Outlook lets you add Holidays to the Outlook calendar, Google Calendar has a built-in feature to add religious holidays and national holidays (or bank holidays) of different countries.
You can add or remove holidays from Google Calendar in these simple steps:
1. Under "Calendars" in the left column, click on the "+" button next to "Other Calendars."
2. Select the "Holiday Calendars" tab (see screenshot)
3. Click on the appropriate "Add Calendar" button.
You can remove a holiday calendar by visiting the same page and clicking on "Remove." The dates are added in a separate calendar that display in different colors (see screenshot)
National and Religious Holidays are added not just for the current year but for all holidays in future years.
http://labnol.blogspot.com/2006/04/add-national-religious-holidays-to.html
You can add or remove holidays from Google Calendar in these simple steps:
1. Under "Calendars" in the left column, click on the "+" button next to "Other Calendars."
2. Select the "Holiday Calendars" tab (see screenshot)
3. Click on the appropriate "Add Calendar" button.
You can remove a holiday calendar by visiting the same page and clicking on "Remove." The dates are added in a separate calendar that display in different colors (see screenshot)
National and Religious Holidays are added not just for the current year but for all holidays in future years.
http://labnol.blogspot.com/2006/04/add-national-religious-holidays-to.html
Friday, May 1, 2009
Widget Gallery
The following are widgets and panels available in the GWT user-interface library. You can view a code example of each widget in the Showcase sample application.
http://code.google.com/webtoolkit/doc/1.6/RefWidgetGallery.html
http://code.google.com/webtoolkit/doc/1.6/RefWidgetGallery.html
Subscribe to:
Posts (Atom)