An Application Programming Interface(API) is often referred to in various contexts making it difficult for non programmers or new programmers to grasp what an API actually is. To make the confusion worse it's meaning has evolved from what it was originally intended to more of a concept or umbrella term. Time to clear up the confusion.
Let me delve into what an API actually is, so no matter your profession you will have a correct and complete understanding of an “API”. We will do this in a few parts. First will get a general but shallow understanding of what an API is. Then we will dissect the word “Application Programming Interface” primarily to understand why it was named such. Finally we take a look at what a real API might look like and even work with a few very simple API's so you have a practical understanding that will be sure to stick (note: anyone can do this you don't need to be a programmer.)
GETTING A GENERAL UNDERSTANDING OF AN API
wiki: An application programming interface (API) is a specification intended to be used as an interface by software components to communicate with each other.
Taylor: An Application Programming Interface is a set of instructions on how one program can be worked with by another outside program.
So what the heck does that mean? Lets clear this up a bit. As a programmer the way we develop big complex programs is by writing lots of little programs and then putting them all together. For example: Lets say the big program is (the evil) Facebook, a little program that we might need to write so we can use it in the bigger program is a “Poke” program.
The “Poke Program” allows one user to poke another user. The user that is poked is then notified that they were poked and may choose to poke back if the wish.
So to complete the Facebook program we need to write lots of other little programs such as a “like program”, “add friend program”, “login program” , “chat program” and so on. We call all these little programs our library of programs or code library. Once we are done we realize that by letting others use some of our little programs we can grow our facebook platform even more. Facebook does just that and documents and allows anyone the ability to use a number of these little programs.
All of the little programs that Facebook lets others use is known as the “Facebook API”.
I will go into how exactly how “anyone has the ability to use” a little facebook program in the section below.
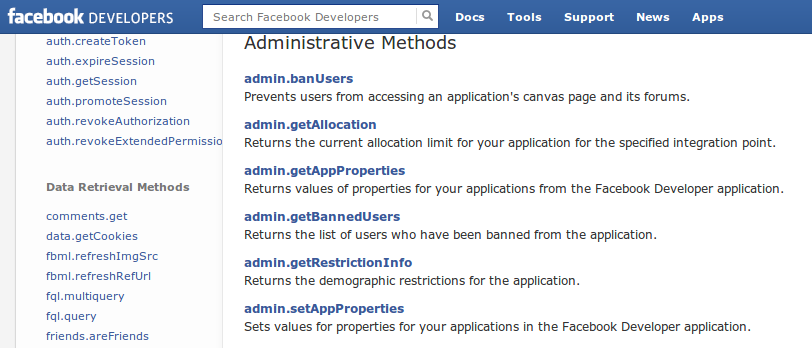
Screen shot of just a few of the “Little Programs” that make up the Facebook API.
BREAKING DOWN THE WORD “APPLICATION PROGRAMMING INTERFACE”
So to understand the word "Application Programming Interface" we need to back up a second and look at how it originated. So before the web was around, when we talked about programming were were generally talking about either System programming (Windows OS) or application programming (software that ran on top of windows OS, calculators, minesweeper, paint etc...). In order for the application programmers to be able to develop their applications they needed to tap into or interface with the Windows operating system. So naturally Windows offered the .Windows Application Programming Interface. - which was a manual on how application programs could tap into the Windows OS.
As the web and many other technologies evolved the term API seemed to stick even for software and technology that was not specific to the "Application Programming" Context. So now the term API is commonly used to refer to any sort of program to program interface. I'd suggest a better name would be "Program to Program Interface" - but after giving "PPI" a google it looks pretty soundly used - maybe we could take over "PTPI" from these guys http://www.ptpi.org/
Another player that helped the term "API" stick was the commonly referred to "Advanced Programming Interface" also acronym-ed "API". The "Advanced Programming Interface" was a manual on how to work with complex pieces of software.
So there we have it the term "API" is born and brought into the modern age. The common misuse of the word becomes widely accepted and "API" becomes the bona fide term for how one program is able to interact with another program. I will keep pushing for the acronym PPI to become widely accepted..... but I wouldn't hold your breath.
REAL LIFE USAGE OF API'S
So now you should generally understand what an API is, but may not really understand how one can work with or use an “API”. Let's take the facebook API and discuss in detail what it may look like and how one can work with it. So for all of the little programs that make up the Facebook API: “like program”, “add friend program”, “login program” , “chat program”, “poke program” facebook will have a snibbit of documentation. (Every API documentation looks a little different but generally try to follow some common format shown below.)
Lets look at what the “Poke Program” documentation may look like this
NOTE: THIS IS NOT A REAL API JUST AN EXAMPLE
Program name : Poke
Details: “Poke” allows one user to poke another user. The user that is poked is notified of the poke and may choose to poke back.
Usage: When being used the little poke program needs to know the id of the user who is doing the poking and the id of the user who is getting poked.
Description: Poke program may be accessed over http at the following url, the “from_poker” parameter should be a integer and the “to_poker” should be and integer.
Url: http://www.facebook.com/api/poke?from_poker=123&to_poker=234
So if (this were real and) you went to the following url in your browser “http://www.facebook.com/api/poke?from_poker=123&to_poker=234” you would have just made user “123” poke user “234” - Congrats. We will do some real examples in a second.
So this is an example of accessing an API over the web, this is how most API's today are accessed and will generally be what people are talking about when they say “API”. Fun fact - API's that are accessed over the web are called “Web Services” and use the HTTP protocol.
The cool thing about these API's is that non programmers could potentially work with them (and we will in a moment) as all they have to do is type a URL with some particular parameters into a browser.
There are also other ways of working with API's that require one to write code, but the principle is the same: there is some little program written by party 1 that is now letting party 2 use that program. Example: The “poke program” may also be accessed in some programming language by including the “facebook code library” and then calling the little poke program.
Include facebook_code_library;
call facebook_poker(123,234);
This piece of code has the same effect as going to the Facebook “Poke URL”, we simply used a different protocol to work with the API.
So now you should have a good understanding of what an API is even how to work with one. In order to not dumb people down it's worth mentioning that not all API's are accessible over the web, some may only be accessible by using a particular programming language. The windows operation system for example has an API that is very confusing and only accessible through programming languages known by the uberist off Geeks.
REAL LIFE API EXAMPLES
And now the fun stuff - lets look at few Web API's so we can get a feel for how they are used.
First lets take look at this awesome “Zip Code Catcher API” built by some unknown stud developer.
Here is the documentation:
Name: Zip Code Catcher
Description: Lets a user download all the zip codes that lie within a given circle.
Url Parameters:
shape: circle (may be able to take in additional shapes in the future)
center: Is a [lat,lng] points on the earth radius: Is the radius of the circle given in meters.
API Url: http://www.woodstitch.com/rest/zip_codes.php?shape=circle¢er=[34.08,-118.26]&radius=17455
If you hit that example URL the “Zip Code Catcher” will download all the zip codes within a 17,455m (ruffly 10 mile) radius of the center point [34.08,-118.26] which just so happens to be Los Angeles. You can change the center or the radius to get different results - pretty cool huh.
Here is another cool API that you could use, that allows a user to give a keyword topic as a parameter and then outputs a paragraph of text about that topic. If you want to build your own API just snag some web hosting from this list and start coding out.
Name: Keyword to Paragraph tool
Description: lets the user input a keywords and returns a unique paragraph of content about that keyword
URL Parameters:
pass: “allowme”
keyword: any keyword that the user want a topic on
API URL: http://assets.giverose.com/contentspinner/get_topic_content.php
Example: http://assets.giverose.com/contentspinner/get_topic_content.php?pass=openup&topic=big bird
So hit that url and you will see that it gives you a paragraph of unique content about big bird. Pretty Cool huh!
Note: Some API's such as this one require a password, this gives the person who owns the API control over how the want other to use their programs.
I trust that no one will use this API for SPAM or any unethical and shady business practices... O wait thats the only things it's good for. What hack is building these tools anyway 

I hope after reading this you have a better understanding of what an API is, how they work and are used in day to day business. Questions, comments, feedback and suggestions are welcomed.
No comments:
Post a Comment